![[C언어] typedef로 자료형 재정의하기](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2Fbm6mwM%2Fbtsakib3hHo%2FuATWHTIlKa6XizpnPXVXXk%2Fimg.jpg)
[C언어] typedef로 자료형 재정의하기프로그래밍 언어/C2023. 4. 14. 23:24
Table of Contents
typedef
는 기존 자료형에 새로운 이름을 부여해 재정의할 수 있게 하는 C언어의 키워드이다. 프로그램의 가독성을 높이고 시스템 간 호환성을 개선하기 위한 용도로 사용된다.
typedef int x; // x를 int와 동일한 자료형으로 정의
아래와 같이 복잡한 자료형에 의미있는 이름을 부여할 수 있다.
typedef unsigned long long int uint64;
시스템에 따라 자료형의 크기가 다를 수 있으므로 프로그램의 시스템 간 호환성을 위해 typedef
로 추상화할 수 있다.
#ifdef _WIN32
typedef int int32_t;
#else
typedef long int32_t;
endif
구조체 자료형 재정의
구조체와 typedef
를 함께 사용해서 간결하고 가독성 있게 만들 수 있다.
typedef struct {
int x;
int y;
} Point;
Point p; // struct Point p; 대신 사용 가능
자기 참조 구조체
자기 참조 구조체Self−Referential Structure는 자신과 동일한 타입의 구조체를 가리키는 포인터를 멤버로 포함하는 구조체이다.
연결 리스트, 트리, 그래프 등과 같은 동적 데이터 구조를 구현할 때 주로 사용된다.
typedef struct Node {
int data; // 데이터 필드
struct Node* next; // 다음 노드를 가리키는 포인터
} Node;
구조체 정의 내에서 완전한 구조체 타입은 사용할 수 없다.
typedef struct Node {
int data;
Node* next; // Error
} Node;
구조체 태그를 사용해서 포인터를 선언한다.
typedef struct Node {
int data;
struct Node* next; // Good!
} Node;
typedef
로 정의한 Node
를 가지고 아래와 같은 함수들도 생성할 수 있다.
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
void insertNode(Node** head, int data) {
Node* newNode = createNode(data);
newNode->next = *head;
*head = newNode;
}
void printList(Node* head) {
Node* current = head;
while (current != NULL) {
printf("%d -> ", current->data);
current = current->next;
}
printf("NULL\n");
}
실행 가능한 전체 코드는 아래와 같다.
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node *next;
} Node;
Node *createNode(int data) {
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
void insertNode(Node **head, int data) {
Node *newNode = createNode(data);
newNode->next = *head;
*head = newNode;
}
void printList(Node *head) {
Node *current = head;
while (current != NULL) {
printf("%d -> ", current->data);
current = current->next;
}
printf("NULL\n");
}
int main() {
Node *head = NULL; // Node 선언 및 초기화
// 노드 삽입
insertNode(&head, 3);
insertNode(&head, 7);
insertNode(&head, 12);
insertNode(&head, 9);
// 리스트 출력
printf("연결 리스트: ");
printList(head);
// 새 노드 추가
insertNode(&head, 15);
// 새 리스트 출력
printf("갱신된 연결 리스트: ");
printList(head);
// 메모리 해제
Node *current = head;
while (current != NULL) {
Node *temp = current;
current = current->next;
free(temp);
}
}
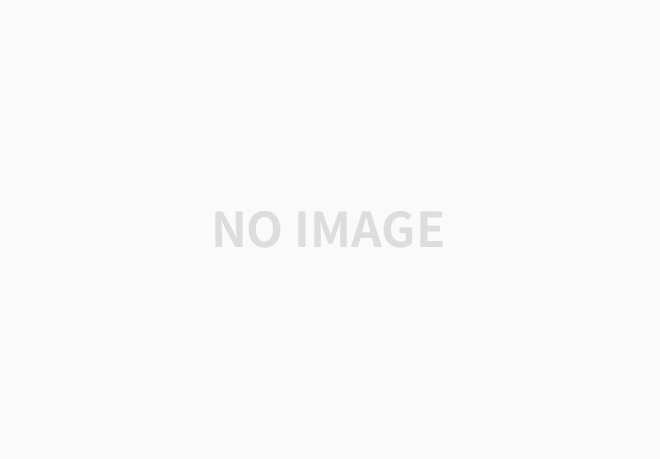
728x90
반응형
'프로그래밍 언어 > C' 카테고리의 다른 글
[C언어] 문자와 문자열 (0) | 2024.07.14 |
---|---|
[C언어] union으로 공용체 정의하기 (0) | 2023.04.14 |
[C언어] struct로 구조체 정의하기 (0) | 2023.04.14 |
[C언어] 배열 포인터와 포인터 배열 (1) | 2023.04.14 |
[C언어] 배열 파라미터와 포인터 파라미터의 차이점 (0) | 2023.04.14 |
@junyeokk :: 나무보다 숲을
컴퓨터 전공 관련, 프론트엔드 개발 지식들을 공유합니다. React, Javascript를 다룰 줄 알며 요즘에는 Typescript에도 관심이 생겨 공부하고 있습니다. 서로 소통하면서 프로젝트 하는 것을 즐기며 많은 대외활동으로 개발 능력과 소프트 스킬을 다듬어나가고 있습니다.
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!